Buffer Over Flow: A Comprehensive Guide with Real-World Examples
One of the oldest and most critical flaws in software security is buffer overflows. The second most exploited vulnerability in 2023 was a heap buffer overflow. It has made innumerable attacks possible over the years, frequently with dire repercussions, like Cloudbleed in 2017. Buffer overflows remain a serious issue despite improvements in security procedures, particularly in software developed in low-level languages like C and C++. Buffer overflow vulnerabilities can be identified early in development by using methods like static analysis and fuzz testing, and they can be avoided altogether by following secure coding best practices.Â
Content
What is Buffer Overflow?
Types of Buffer Overflow
Real-World Examples of Buffer Overflow
Why Buffer Overflow is Dangerous?
How Attackers Exploit Buffer Overflow?
Buffer Overflow Mitigation Techniques
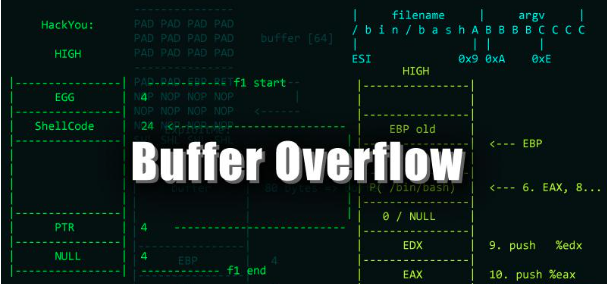
What is Buffer Overflow?
When a program writes more data to a buffer than it can store, this is known as a buffer overflow. Fixed-size memory storage spaces called buffers are used to temporarily store data. The extra data overflows into nearby memory locations when the amount of data exceeds the allotted space, perhaps erasing previously stored information. Unpredictable consequences, such as crashes, data corruption, and the execution of malicious code, may result from this behavior.
Code Example: Simple Buffer Overflow
#include <stdio.h>
#include <string.h>
void vulnerable_function() {
    char buffer[8]; // Buffer can hold only 8 characters
    printf(“Enter some text: “);
    gets(buffer); // Unsafe function, does not check input length
    printf(“You entered: %s\n”, buffer);
}
int main() {
    vulnerable_function();
    return 0;
}
Types of Buffer Overflow
- Buffer Overflow Based on Stacks
The stack memory, which houses local variables and function call data, is where this kind of overflow happens. Attackers can reroute the program’s execution to malicious code and overwrite a function’s return address by overflowing a buffer in the stack.
Â
- Buffer Overflow Based on Heaps
The heap, the dynamically allocated memory space utilized for objects and data structures, is the objective of this type. Attackers can run arbitrary code or alter data structures by taking advantage of a heap-based overflow.
Â
Real-World Examples of Buffer Overflow
- The 1988 Morris Worm
One of the first and most well-known instances of buffer overflow exploitation is the Morris Worm. It spread throughout networks by taking advantage of a buffer overflow vulnerability in the Unix finger service. This worm caused extensive disruption by infecting about 10% of the internet, which was a substantial percentage at the time.
Â
- The 2014 film Heartbleed
A buffer overflow in the TLS heartbeat extension was exploited by Heartbleed, a serious OpenSSL vulnerability. Passwords, usernames, and private keys are among the confidential information that an attacker may obtain from a server’s memory. Millions of websites were impacted by this vulnerability, including well-known ones like Yahoo and Google. - Code Execution for Microsoft Windows (2019)
CVE-2019-0708, also referred to as Blue Keep, was a buffer overflow vulnerability in Windows Remote Desktop Protocol (RDP) that gave attackers the ability to remotely run arbitrary code. Microsoft issued fixes for out-of-date operating systems like Windows XP to stop a possible worm able vulnerability since Blue Keep was so serious.
Â
- Vulnerabilities in Adobe Flash
Over the years, Adobe Flash Player, which is well-known for its security problems, experienced multiple buffer overflow vulnerabilities. These flaws were regularly used to hack user systems and run malicious malware.
Â
Why Buffer Overflow is Dangerous
Particularly risky are buffer overflow vulnerabilities because they can:
Allow Arbitrary programs Execution: This gives attackers the ability to take over the target machine by injecting and running malicious programs.
Cause Denial of Service (DoS): Services may be interrupted by programs crashing or becoming unstable.
Facilitate Privilege Escalation: By taking advantage of a buffer overflow, an attacker can obtain more elevated privileges, including administrator or root access.
Data Integrity Compromise: Unpredictable behavior can result from overwritten memory corrupting important data.
Â
How Attackers Exploit Buffer Overflow
Step 1: Determine Which Program Is at Risk
Attackers look for applications with buffer overflow vulnerabilities using fuzzing or reverse engineering.
Create Malevolent Input in Step Two
In order to carry out harmful commands, attackers generate input that is larger than the buffer’s capacity and include payloads like shellcode.
Step 3: Memory Overwrite
Attackers can rewrite important memory locations, including function pointers or the return address, by providing the malicious data.
Step 4: Execution Redirect
Attackers gain control of the system when the program’s execution is redirected to the malicious payload by the rewritten memory.
Â
Buffer Overflow Mitigation Techniques
- Validation of Input
Verify the length of the incoming data before processing to make sure it doesn’t exceed buffer sizes.
2. Secure Features
Steer clear of dangerous methods like C’s gets() and use secure ones like strncpy() instead of strcpy().
3. Protections for Compilers
Features of contemporary compilers include:
Stack Canaries: To identify overflows, special values are positioned in between buffers and control data.
By randomly allocating memory addresses, Address Space Layout Randomization (ASLR) makes it more difficult for attackers to anticipate where memory will be located.
Â
- Programming Languages That Don’t Use Memory
Buffer overflows are less likely in languages like Python, Java, and Rust since they automatically manage memory and carry out bounds checking.
5. Frequent Repairs
Update your software with the most recent security updates to fix known vulnerabilities.